Initiate connection
Create the Central Manager object
To initiate and set the delegate to the central manager object, confirm PGCentralManagerDelegate
and use the initWithDelegate:enableRestoration:
method.
#import <ConnectSDK/ConnectSDK.h> ... @interface ViewController () <PGCentralManagerDelegate> @property PGCentralManager *central; ... - (void)setup { self.central = [[PGCentralManager alloc] initWithDelegate:self enableRestoration:NO]; }
import ConnectSDK ... class ViewController: UIViewController, PGCentralManagerDelegate { ... var central: PGCentralManager? ... func setup() { central = PGCentralManager(delegate: self, enableRestoration: false) }
Initiate new connections
To initiate a new connection, create a connection QR code by invoking the PGCentralManager
method initiateScannerConnectionWithImageSize
(make sure Bluetooth is on by checking the PGCentralManager
state).
if (self.central.state != PGManagerStatePoweredOn) { // In case Bluetooth is unavailable return; } CGFloat imageSize = MIN(self.qrImageView.frame.size.width, self.qrImageView.frame.size.height); UIImage *image = [self.central initiateScannerConnectionWithImageSize:CGSizeMake(imageSize, imageSize)]; self.qrImageView.image = image;
guard central.state == .poweredOn else { // In case Bluetooth is unavailable return } let imageSize = min(qrImageView.frame.size.width, qrImageView.frame.size.height) let image = central.initiateScannerConnection(withImageSize: CGSize(width: imageSize, height: imageSize)) qrImageView.image = image
Pairing Barcode - PGPairingQR
This feature enables the users of INSIGHT Mobile (iOS) and (watchOS) SDK to generate pairing barcodes based on the provided advertising name. After the barcode is generated in such a way, it can be printed and attached to the Connectivity device so ProGlove scanners can be paired and used.
/// Generates a QR code with the given identifier and error correction level at the given size /// - Parameters: /// - indicator: The advertising name that will be embedded into connection barcode. (e.g "AAAAA"). /// - errorCorrectionLevel: The error correction level /// - size: The size of the image to generate /// - scale: The destination screen points to pixels scale /// - Returns: The generated QR code @objc public static func generate(_ indicator: String, _ errorCorrectionLevel: PGPairingQRGeneratorErrorCorrectionLevel, _ size: CGSize, _ scale: CGFloat) -> UIImage
Generate a connection barcode with additional configurations
You can embed additional custom configurations into the connection barcode. In order to create a connection barcode with an embedded configuration, PGPairingQR class provides methods for generating a pairing QR image, accepting indicators, options, and error object values. As a result, an image containing the connection barcode will be generated. In case of invalid parameters, the result will be NULL and the error parameter will be populated.
Available barcode configuration options - PGPairingQRGeneratorOptions:
fullDiscoveryHandshakeEnabled - A value indicating that the full BLE discovery handshake can be enabled/disabled. If set to false (NO) the advertising name will be excluded from the BLE GATT scan response. The default value is true (YES).
Note
If the device was previously discovered/connected and the fullDiscoveryHandshakeEnabled is set to false, the iOS device will discover a scanner with the previous advertisement name due to the caching mechanism and not be able to connect. In order to prevent this, the BLE should first be disabled and enabled again on the iOS device, so the cache is cleared and the device is able to connect. This should occur only the first time after changing the value to false and when connecting to a device that was previously discovered/connected.
When disabled, the indicator (advertisement name) must not be longer than 8 characters.
imageScale - A value indicating the destination screen points to pixels scale. The default value matches main screen scale.
errorCorrectionLevel - A value indicating the connection barcode correction level. The default value is M.
imageSize - A value indicating the image size. The default value is 300 pixels x 300 pixels.
Connection state machine
The diagram below details the states during the scanner's connection process to the customer app.
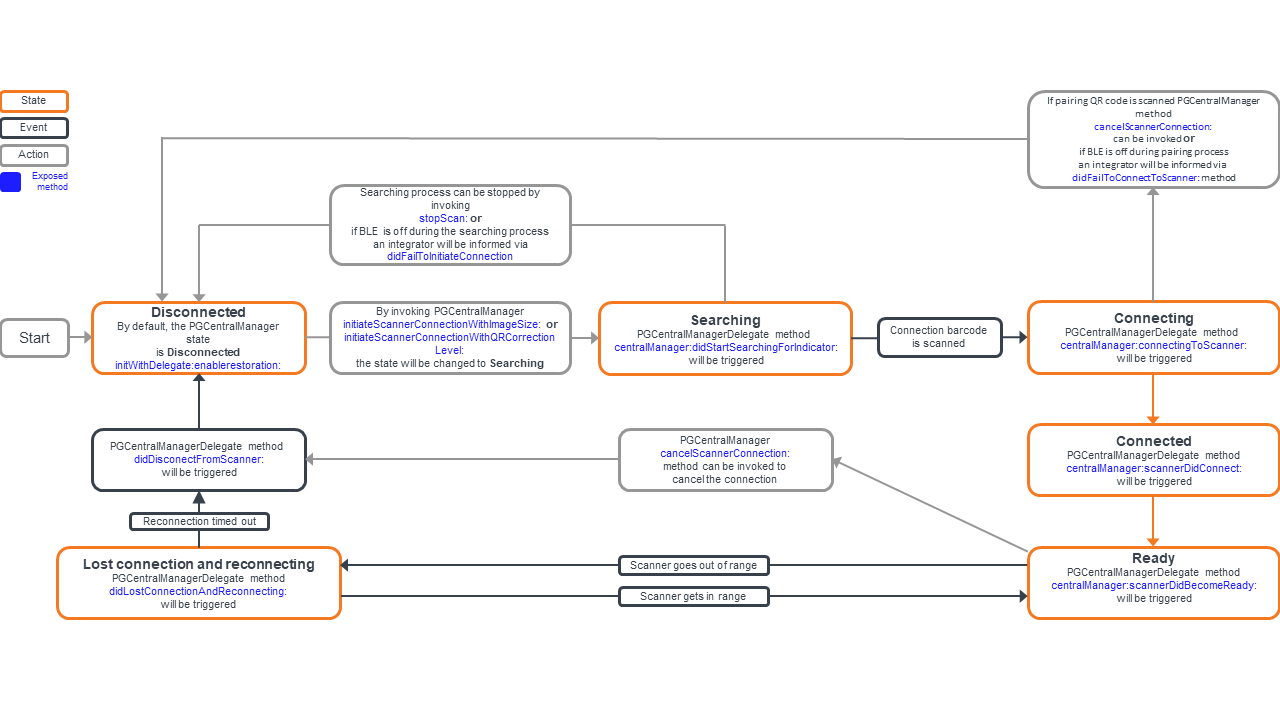