Queueing behavior
When setting screens, requesting device information, or performing any other action on a scanner, the ConnectSDK queues the commands it will send to the device.
For example, if you quickly call, setScreen
3 times successively, the 3 screens will be displayed one after another.
You can cancel all commands currently waiting in the queue and place the next command as the first one to be executed.
Queueing behavior without replace-queue:
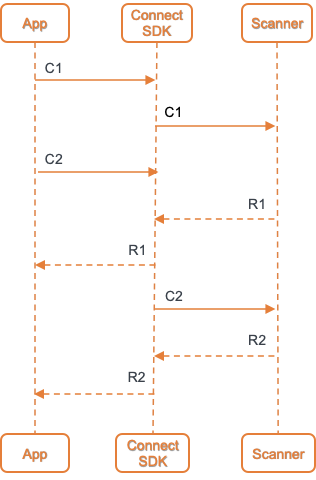
Queueing behavior with replace-queue:
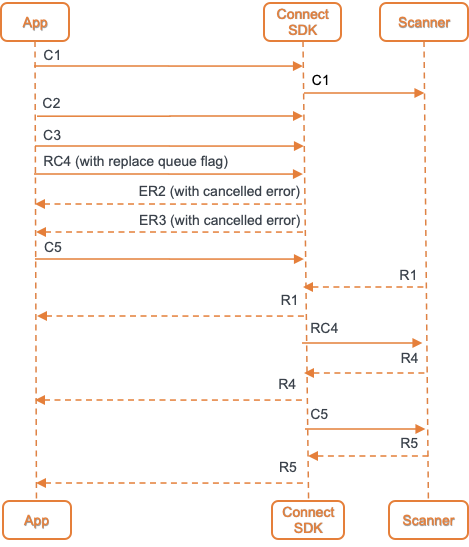
Legend | |
---|---|
Cx | A command triggered via SDK |
Rx | A response (error or result object) to the Cx command |
RCx | A command with the replace-queue flag active |
ERx | An error response to Cx command |
This queue is currently limited to 5 commands. If a command is triggered and there are already 5 commands waiting in the queue, the response is immediate and indicates a full queue.
SDK
When using the ConnectSDK, every command is sent via a PGCommand
object. PGCommand
is used to initiate specific requests to the scanner, it holds the necessary command data and can hold a PGCommandParams
object.
The PGCommandParams
object holds the data on how this command should be processed.
See below for the requests currently available and instructions on how to trigger the command and replace the queue flag.
PGDeviceInformationRequest
PGFeedbackRequest
PGScreenData
PGSetDisplayOrientationRequest
PGConfigurationProfile
PGCaptureImageRequest
To trigger the command with the replace queue flag, add a PGCommandParams
object with replaceQueue
set to true to the PGCommand
.
All commands cancelled while waiting in the queue for execution will result in a call back with the error with domain PGWearableApiErrorDomain
and error code PGWearableApiErrorRequestCanceled
of the PGWearableApiError
.
See below for an example of how to trigger feedback immediately, cancelling all queued commands:
PGCommandParams *commandParams = [[PGCommandParams alloc] init]; commandParams.replaceQueue = YES; PGCommand *command = [[PGCommand alloc] init:[[PGDeviceInformationRequest alloc] init] withParams:commandParams]; [centralManager.connectedScanner requestDeviceInformationWithDeviceInfoCommand:command completionHandler:^(PGDeviceInformation * _Nullable deviceInformation, NSError * _Nullable error) { // Handle response }];
PGCommandParams *commandParams = [[PGCommandParams alloc] init]; commandParams.replaceQueue = YES; PGCommand *command = [[PGCommand alloc] init:[[PGDeviceInformationRequest alloc] init] withParams:commandParams]; [centralManager.connectedScanner requestDeviceInformationWithDeviceInfoCommand:command completionHandler:^(PGDeviceInformation * _Nullable deviceInformation, NSError * _Nullable error) { // Handle response }];
Possible compiler issue

The compiler will complain about the breaking change. The reason is that the PGCommand
type is not generic anymore and has to accept a specific type within the initializer.

The solution to the error is easily fixable by expanding the error message and selecting the Fix
command.
