Initiate connection
Create the Central Manager object
To initiate and set the delegate to the central manager object, confirm PGCentralManagerDelegate
and use the initWithDelegate:enableRestoration:
method.
Example 49. Objective C
#import <ConnectSDK/ConnectSDK.h> ... @interface ViewController () <PGCentralManagerDelegate> @property PGCentralManager *central; ... - (void)setup { self.central = [[PGCentralManager alloc] initWithDelegate:self enableRestoration:NO]; }
Example 50. Swift
import ConnectSDK ... class ViewController: UIViewController, PGCentralManagerDelegate { ... var central: PGCentralManager? ... func setup() { central = PGCentralManager(delegate: self, enableRestoration: false) }
Initiate new connections
To initiate a new connection, create a connection QR code by invoking the PGCentralManager
method initiateScannerConnectionWithImageSize
(make sure Bluetooth is on by checking the PGCentralManager
state).
Example 51. Objective C
if (self.central.state != PGManagerStatePoweredOn) { // In case Bluetooth is unavailable return; } CGFloat imageSize = MIN(self.qrImageView.frame.size.width, self.qrImageView.frame.size.height); UIImage *image = [self.central initiateScannerConnectionWithImageSize:CGSizeMake(imageSize, imageSize)]; self.qrImageView.image = image;
Example 52. Swift
guard central.state == .poweredOn else { // In case Bluetooth is unavailable return } let imageSize = min(qrImageView.frame.size.width, qrImageView.frame.size.height) let image = central.initiateScannerConnection(withImageSize: CGSize(width: imageSize, height: imageSize)) qrImageView.image = image
Connection state machine
The diagram below details the states during the scanner's connection process to the customer app.
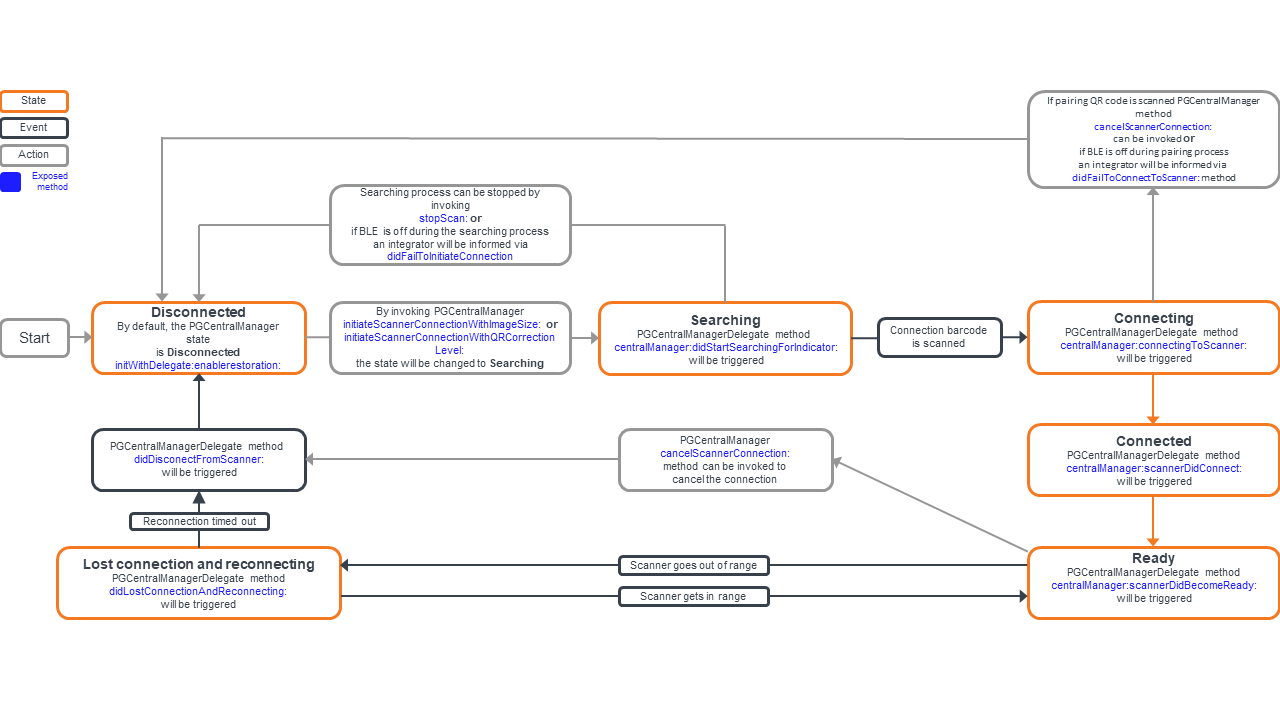